Canvas API
The Canvas API provides a means for drawing graphics via JavaScript and the HTML <canvas> element.
14th August 2022
Time to read: 1 min
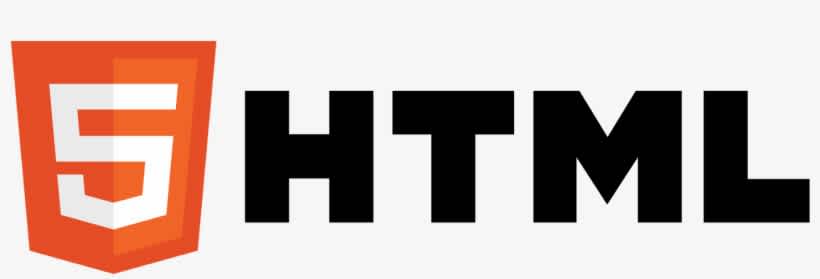
The Canvas API largely focuses on 2D graphics. The WebGL API, which also uses the
<canvas>
element, draws hardware-accelerated 2D and 3D graphics.
Basic example
This simple example draws a green rectangle onto a canvas.
HTML
<canvas id="canvas" width="250" height="200"></canvas>
When no width and height attributes are specified, the canvas will initially be 300 pixels wide and 150 pixels high. CSS can also be used, but the aspect ratio should be respected to avoid distortion.
The
<canvas>
element can be styled just like any normal image (margin, border, background…).
JavaScript
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
ctx.fillStyle = 'green';
ctx.fillRect(10, 10, 150, 100);
Fallback content
Fallback content should always be defined, to be displayed in older browsers not supporting canvas
, like versions of Internet Explorer earlier than version 9 or textual browsers.
<canvas id="clock" width="150" height="150">
<img src="images/clock.png" width="150" height="150" alt="clock image"/>
</canvas>
Note:
</ canvas>
closing tag is required.
Checking for support
const canvas = document.getElementById('canvas');
if (canvas.getContext) {
const ctx = canvas.getContext('2d');
// drawing code here
} else {
// canvas-unsupported code here
}
A basic template
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="utf-8"/>
<title>Canvas tutorial</title>
<script>
function draw() {
const canvas = document.getElementById('canvas');
if (canvas.getContext) {
const ctx = canvas.getContext('2d');
}
}
</script>
<style>
canvas { border: 1px solid black; }
</style>
</head>
<body onload="draw();">
<canvas id="canvas" width="150" height="150">
<!-- Fallback content here -->
</canvas>
</body>
</html>
A simple example
<!DOCTYPE html>
<html lang="en-US">
<head>
<meta charset="UTF-8"/>
<title>Canvas experiment</title>
<script type="application/javascript">
function draw() {
const canvas = document.getElementById('canvas');
if (canvas.getContext) {
const ctx = canvas.getContext('2d');
ctx.fillStyle = 'rgb(200, 0, 0)';
ctx.fillRect(10, 10, 50, 50);
ctx.fillStyle = 'rgba(0, 0, 200, 0.5)';
ctx.fillRect(30, 30, 50, 50);
}
}
</script>
</head>
<body onload="draw();">
<canvas id="canvas" width="150" height="150">
<!-- Fallback content here -->
</canvas>
</body>
</html>
Result
Related pages:
Canvas Shapes
Draw rectangles, triangles, lines, arcs and curves.
Canvas Styles and Colours
Add different colors, line styles, gradients, patterns and shadows to canvas elements.