Gatsby Plugin Google Analytics
Easily add Google Analytics to your Gatsby site.
8th July 2022
Time to read: 1 min
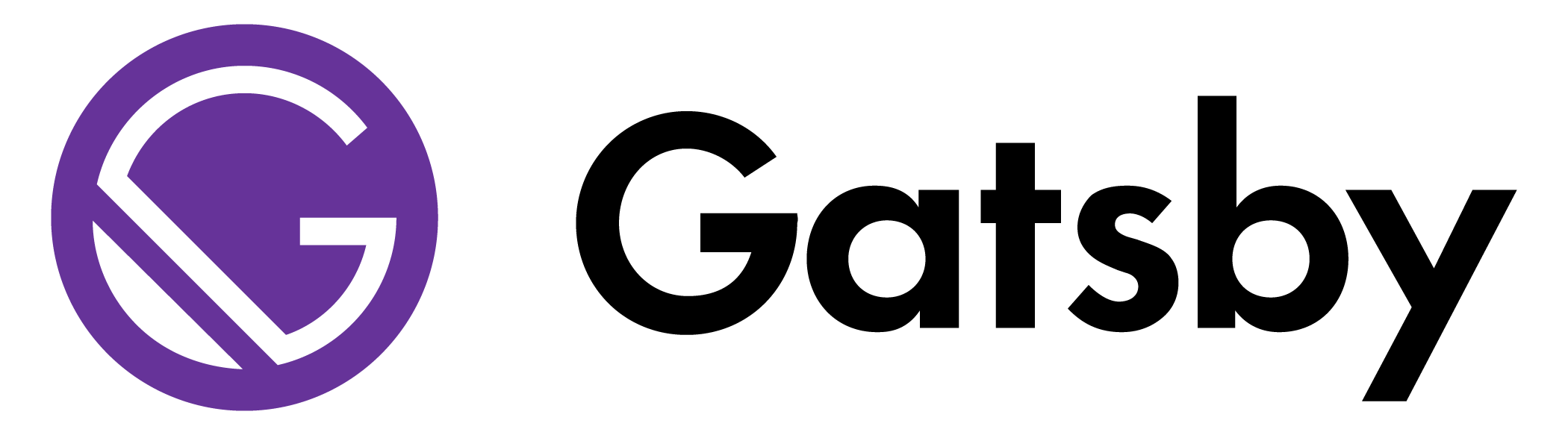
Install
npm install gatsby-plugin-google-analytics
How to use
gatsby-config.js
plugins: [
// Make sure this plugin is first in the array of plugins
{
resolve: `gatsby-plugin-google-analytics`,
options: {
trackingId: "UA-XXXXXXXXXX-XX",
head: true,
enableWebVitalsTracking: true,
},
},
]
Note that this plugin is disabled while running gatsby develop
. This way, actions are not tracked while you are still developing your project. Once you run gatsby build
the plugin is enabled. Test it with gatsby serve
.
Options
// In your gatsby-config.js
module.exports = {
plugins: [
{
resolve: `gatsby-plugin-google-analytics`,
options: {
// The property ID; the tracking code won't be generated without it
trackingId: "YOUR_GOOGLE_ANALYTICS_TRACKING_ID",
// Defines where to place the tracking script - `true` in the head and `false` in the body
head: false,
// Setting this parameter is optional
anonymize: true,
// Setting this parameter is also optional
respectDNT: true,
// Avoids sending pageview hits from custom paths
exclude: ["/preview/**", "/do-not-track/me/too/"],
// Delays sending pageview hits on route update (in milliseconds)
pageTransitionDelay: 0,
// Enables Google Optimize using your container Id
optimizeId: "YOUR_GOOGLE_OPTIMIZE_TRACKING_ID",
// Enables Google Optimize Experiment ID
experimentId: "YOUR_GOOGLE_EXPERIMENT_ID",
// Set Variation ID. 0 for original 1,2,3....
variationId: "YOUR_GOOGLE_OPTIMIZE_VARIATION_ID",
// Defers execution of google analytics script after page load
defer: false,
// Any additional optional fields
sampleRate: 5,
siteSpeedSampleRate: 10,
cookieDomain: "example.com",
// defaults to false
enableWebVitalsTracking: true,
},
},
],
}
Track Custom Event
To allow custom events to be tracked, the plugin exposes a function to include in your project.
To use it, import the package and call the event within your components and business logic.
import React from "react"
import { trackCustomEvent } from "gatsby-plugin-google-analytics"
const Component = () => (
<div>
<button
onClick={e => {
// To stop the page reloading
e.preventDefault()
// Lets track that custom click
trackCustomEvent({
// string - required - The object that was interacted with (e.g.video)
category: "Special Button",
// string - required - Type of interaction (e.g. 'play')
action: "Click",
// string - optional - Useful for categorizing events (e.g. 'Spring Campaign')
label: "Gatsby Plugin Example Campaign",
// number - optional - Numeric value associated with the event. (e.g. A product ID)
value: 43,
})
//... Other logic here
}}
>
Tap that!
</button>
</div>
)
export default Component
All Fields Options
category
: string - requiredaction
: string - requiredlabel
: stringvalue
: integernonInteraction
: booltransport
: stringhitCallback
: function
For more information see the Google Analytics documentation.