React and Phaser.js
How to add Phaser.js to your React project.
27th August 2022
Time to read: 1 min
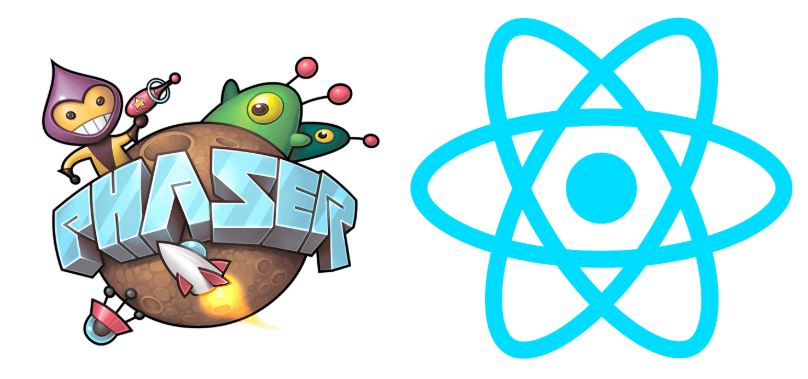
Install Phaser.js
Install via npm
:
npm install phaser
Create custom hook
// /hooks/usePhaser.js
import { useState, useEffect } from 'react'
import Phaser from 'phaser'
export function usePhaser (config, containerRef) {
const [game, setGame] = useState()
useEffect(() => {
const resize = () => {
window.location.reload()
}
if (!game && containerRef.current) {
config.width = window.innerWidth
config.height = window.innerHeight
const newGame = new Phaser.Game({ ...config, parent: containerRef.current })
setGame(newGame)
} else if (game) {
window.addEventListener("resize", resize, true)
}
return () => {
game?.destroy(true)
window.removeEventListener("resize", resize, true)
}
}, [config, containerRef, game])
return game
}
Create Game
Add usePhaser
custom hook to game component:
import React, { useRef } from 'react'
import Phaser from 'phaser'
import { usePhaser } from "@hooks/";
const MyGame = () => {
// reference to game container div:
const parentEl = useRef()
// add game functions here:
const someFunction = (x, y) => {
return x + y
}
// create your game here:
class Game extends Phaser.Scene {
constructor ()
{
super('Game');
}
preload ()
{
}
create ()
{
}
update ()
{
}
}
// create config:
const config = {
type: Phaser.AUTO,
parent: 'phaser-example',
scale: {
mode: Phaser.Scale.RESIZE,
},
backgroundColor: '#111',
physics: {
default: 'arcade',
arcade: {
gravity: {y:0,x:0},
}
},
scene: [Game]
};
usePhaser(config, parentEl)
return (
<div ref={parentEl} />
)
}
export default MyGame
Add component element
Import Suspense
from React
and import the game component using lazy loading:
import React, { Suspense } from "react"
import Loading from '@components/loading'
const MyGame = React.lazy(() => import('@components/MyGame'))
Add the component element in your jsx
:
<Suspense fallback={<Loading />}>
<MyGame />
</Suspense>