Gatsby Plugin Twitter
Loads the Twitter JavaScript for embedding tweets, timelines, share and follow buttons. Lets you add tweets to markdown and in other places.
17th July 2022
Time to read: 1 min
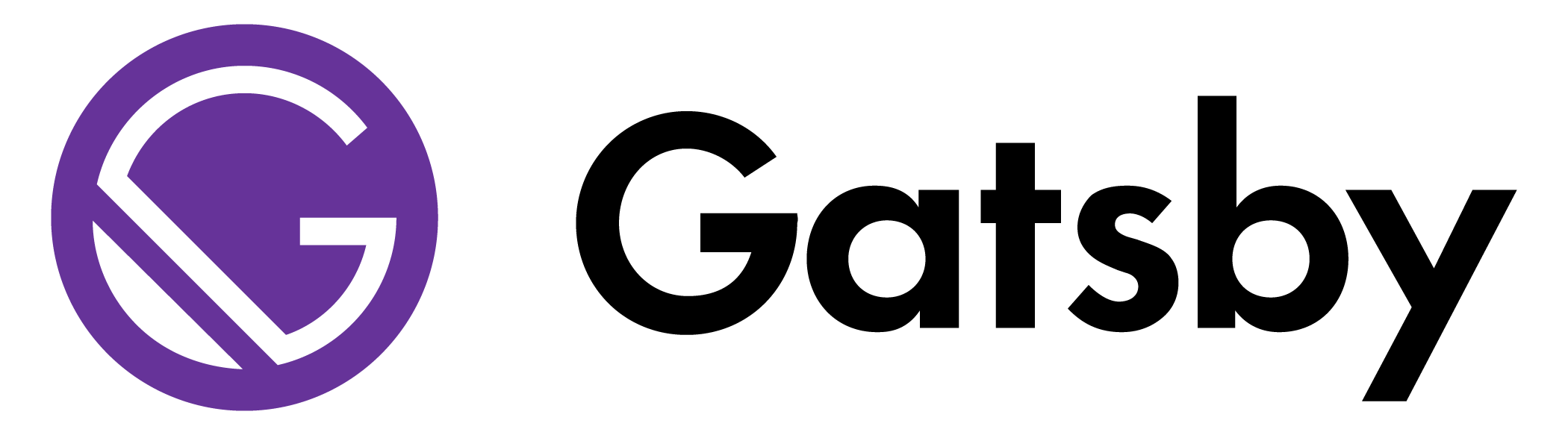
Install
npm install gatsby-plugin-twitter
Configure
// In your gatsby-config.js
plugins: [`gatsby-plugin-twitter`]
Create Component
Create a folder called TwitterEmbed
in your components
folder. In this folder create index.js
.
// index.js
export * from './TwitterEmbed';
export { default } from './TwitterEmbed';
In the same folder create the component:
// TwitterEmbed.js
import React from 'react'
const TwitterEmbed = ( {handle, limit} ) => {
const href = "https://twitter.com/" + handle
return (
<div id={handle} className="mb-0 text-center">
<a href={href} data-tweet-limit={limit} data-chrome='noheader nofooter' title='Opens in new tab'>@{handle}</a>
</div>
)
}
const defaultProps = {
limit: 1,
}
export default TwitterEmbed
Add component element
import TwitterEmbed from '../TwitterEmbed'
const handle = 'CineBlogs1'
const limit = 3
return (
<TwitterEmbed handle={handle} limit={limit}></TwitterEmbed>
)