React ES6
React uses ES6, which stands for ECMAScript 6.
30th July 2022
Time to read: 1 min
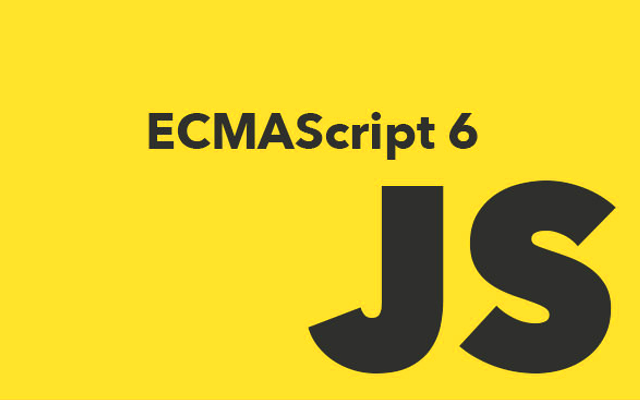
ECMAScript was created to standardize JavaScript, and ES6 is the 6th version of ECMAScript, it was published in 2015, and is also known as ECMAScript 2015.
ES6 Features
Arrow Functions
Arrow functions allow us to write shorter function syntax.
// Arrow Functions return value by default
hello = () => "Hello World!";
// with params
hello = (val) => "Hello " + val;
// if you have only one parameter, you can skip the parentheses as well
hello = val => "Hello " + val;
Variables
There are three ways of defining variables: var
, let
, and const
var
var
has a function scope.
var x = 5.6;
let
let
is the block scoped version of var
, and is limited to the block (or expression) where it is defined.
let x = 5.6;
const
const
is a variable that once it has been created, its value can never change. It does not define a constant value. It defines a constant reference to a value.
const x = 5.6;
Map
In React, map()
can be used to generate lists in your jsx
.
const Articles = ( { posts } ) => {
return (
<ul className={articles}>
{posts && posts.map((post, index) => (
<ArticleSlug key={index} post={post} />
))}
</ul>
)
}
It is necessary to add
key
attribute to list elements.
Destructuring
Arrays
Assign array items to a variable:
const vehicles = ['mustang', 'f-150', 'expedition'];
const [car, truck, suv] = vehicles;
// or
const [car,, suv] = vehicles;
Objects
const vehicleOne = {
brand: 'Ford',
model: 'Mustang',
type: 'car',
year: 2021,
color: 'red'
}
myVehicle(vehicleOne);
function myVehicle({type, color, brand, model}) {
const message = 'My ' + type + ' is a ' + color + ' ' + brand + ' ' + model + '.';
}
Usage in React
const ArticleTemplate = ({ post }) => {
const {title, description, createdAt, body} = post
return (
<h1>{title}</h1>
<p>{description}</p>
<p>{createdAt}</p>
<Markdown mdx={body.childMdx.body} />
)
}
Modules
JavaScript modules allow you to break up your code into separate files. ES Modules rely on the import
and export
statements.
Exports
You can export a function or variable from any file. There are two types of exports: Named and Default.
Named Exports
export const name = "Jesse"
export const age = 40
// or
const name = "Jesse"
const age = 40
export { name, age }
Default Exports
You can only have one default export in a file.
const message = () => {
const name = "Jesse";
const age = 40;
return name + ' is ' + age + 'years old.';
};
export default message;
Import
You can import modules into a file in two ways, based on if they are named exports or default exports. Named exports must be destructured using curly braces. Default exports do not.
Import from named exports
import { name, age } from "./person.js";
Import from default exports
import message from "./message.js";
Logical Operator
Syntax: condition && <expression if true>
authenticated && <LoggedIn />;
Ternary Operator
The ternary operator is a simplified conditional operator like if
/ else
.
Syntax: condition ? <expression if true> : <expression if false>
authenticated ? renderApp() : renderLogin();
Usage in React
The ternary operator may be chained like so:
{
loading ? <h2>Loading...</h2> :
error ? <h1>Error</h1> :
<h1>Results</h1>
}
Spread Operator
The JavaScript spread operator (...) allows us to quickly copy all or part of an existing array or object into another array or object.
Arrays
const numbersOne = [1, 2, 3];
const numbersTwo = [4, 5, 6];
const numbersCombined = [...numbersOne, ...numbersTwo];
Assign the first and second items from numbers
to variables and put the rest in an array:
const numbers = [1, 2, 3, 4, 5, 6];
const [one, two, ...rest] = numbers;
console.log(rest) // 3,4,5,6
Merge
Merge two arrays and remove duplicates:
var myArray1 = [5, 7, 9];
var myArray2 = [9, 8, 5, 10];
var myFinalArray = [...new Set([...myArray1 ,...myArray2])];
console.log(myFinalArray); // [ 5, 7, 9, 8, 10 ]
Objects
Combine two objects:
const myVehicle = {
brand: 'Ford',
model: 'Mustang',
color: 'red'
}
const updateMyVehicle = {
type: 'car',
year: 2021,
color: 'yellow'
}
const myUpdatedVehicle = {...myVehicle, ...updateMyVehicle}