React useState Hook
The React useState Hook allows us to track state in a function component.
4th August 2022
Time to read: 1 min
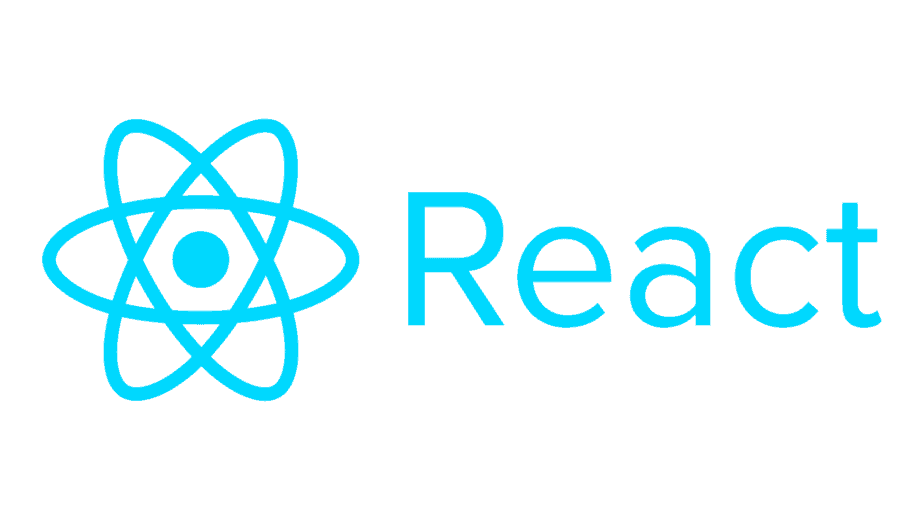
Import useState
import React, {useState} from "react"
Initialize useState
useState
accepts an initial state and returns two values:
- The current state.
- A function that updates the state.
function FavoriteColor() {
const [color, setColor] = useState("");
}
In this case, the initial state to an empty string: useState("")
Read State
function FavoriteColor() {
const [color, setColor] = useState("red");
return <h1>My favorite color is {color}!</h1>
}
Update State
To update state, use the state updater function.
function FavoriteColor() {
const [color, setColor] = useState("red");
return (
<>
<h1>My favorite color is {color}!</h1>
<button
type="button"
onClick={() => setColor("blue")}
>Blue</button>
</>
)
}
What Can State Hold
The useState
Hook can be used to keep track of strings, numbers, booleans, arrays, objects, or any combination of these:
function Car() {
const [brand, setBrand] = useState("Ford");
const [model, setModel] = useState("Mustang");
const [year, setYear] = useState("1964");
const [color, setColor] = useState("red");
return (
<>
<h1>My {brand}</h1>
<p>
It is a {color} {model} from {year}.
</p>
</>
)
}
Or, we can just use one state and include an object instead:
function Car() {
const [car, setCar] = useState({
brand: "Ford",
model: "Mustang",
year: "1964",
color: "red"
});
return (
<>
<h1>My {car.brand}</h1>
<p>
It is a {car.color} {car.model} from {car.year}.
</p>
</>
)
}
Updating Objects and Arrays in State
When state is updated, the entire state gets overwritten.
What if we only want to update the color of our car?
If we only called setCar({color: "blue"})
, this would remove the brand, model, and year from our state.
We can use the JavaScript spread operator to help us.
function Car() {
const [car, setCar] = useState({
brand: "Ford",
model: "Mustang",
year: "1964",
color: "red"
});
const updateColor = () => {
setCar(previousState => {
return { ...previousState, color: "blue" }
});
}
return (
<>
<h1>My {car.brand}</h1>
<p>
It is a {car.color} {car.model} from {car.year}.
</p>
<button
type="button"
onClick={updateColor}
>Blue</button>
</>
)
}