Tailwind Breakpoints
The default breakpoints are inspired by common device resolutions.
4th August 2022
Time to read: 1 min
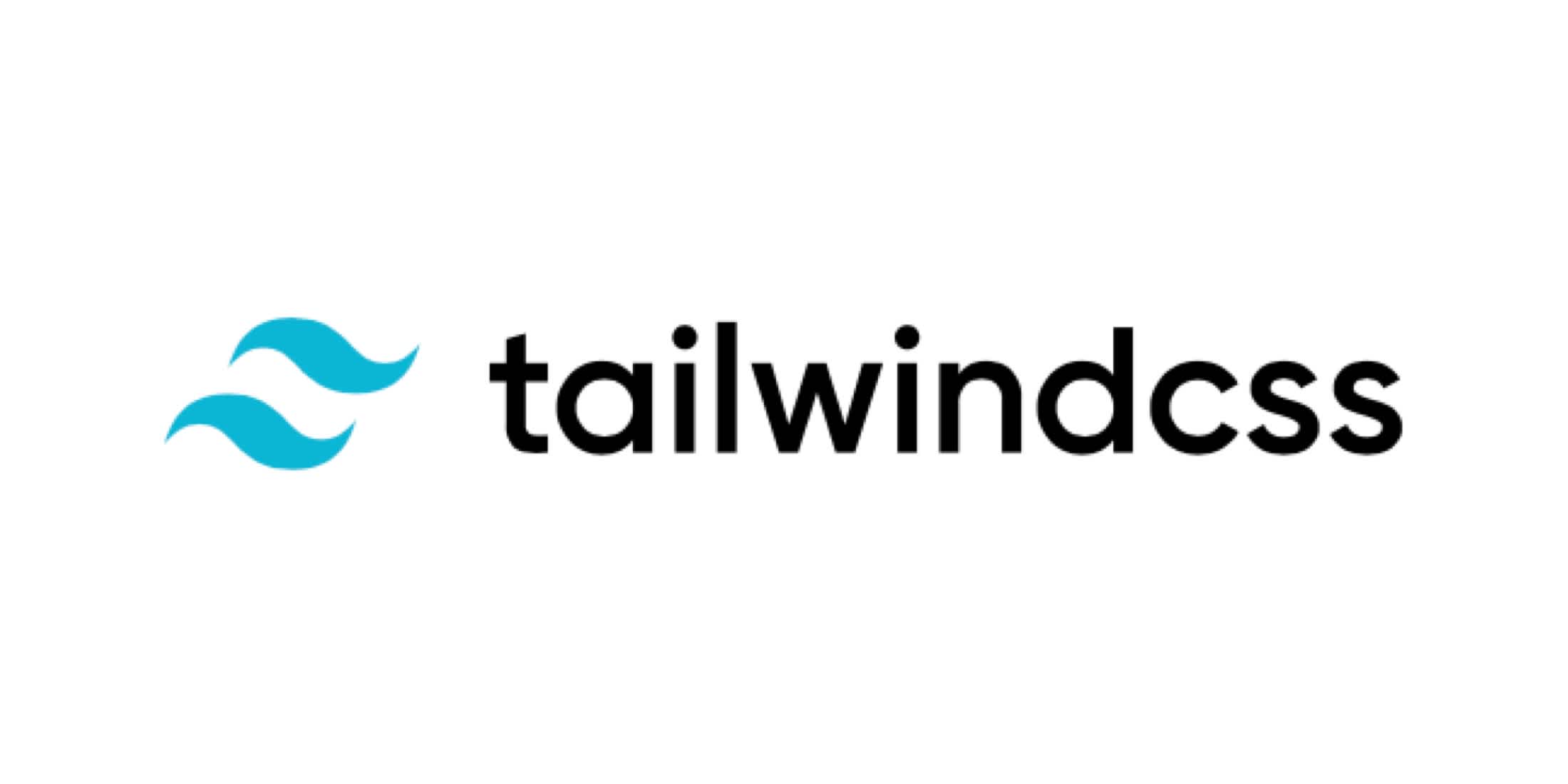
Default Breakpoints
// tailwind.config.js
module.exports = {
theme: {
screens: {
'sm': '640px',
// => @media (min-width: 640px) { ... }
'md': '768px',
// => @media (min-width: 768px) { ... }
'lg': '1024px',
// => @media (min-width: 1024px) { ... }
'xl': '1280px',
// => @media (min-width: 1280px) { ... }
'2xl': '1536px',
// => @media (min-width: 1536px) { ... }
}
}
}
Usage
The values are the min-width where that breakpoint should start.
// tailwind.config.js
<div className='text-center md:text-right lg:text-left'>
Text alignment depends on screen width.
</div>
Overriding the defaults
module.exports = {
theme: {
screens: {
'sm': '576px',
// => @media (min-width: 576px) { ... }
'md': '960px',
// => @media (min-width: 960px) { ... }
'lg': '1440px',
// => @media (min-width: 1440px) { ... }
},
}
}
Any default screens you haven’t overridden (such as xl using the above example) will be removed and will not be available as screen modifiers.
Overriding a single screen
Use the extend
key like so:
// tailwind.config.js
module.exports = {
theme: {
extend: {
screens: {
'lg': '992px',
// => @media (min-width: 992px) { ... }
},
},
},
}
This will replace the default screens value with the same name, without changing the order of your breakpoints.
Adding larger breakpoints
The easiest way to add an additional larger breakpoint is using the extend
key:
// tailwind.config.js
module.exports = {
theme: {
extend: {
screens: {
'3xl': '1600px',
},
},
},
}
Adding smaller breakpoints
Override the entire screens key, re-specifying the default breakpoints:
// tailwind.config.js
const defaultTheme = require('tailwindcss/defaultTheme')
module.exports = {
theme: {
screens: {
'xs': '475px',
...defaultTheme.screens,
},
},
}
Using custom screen names
// tailwind.config.js
module.exports = {
theme: {
screens: {
'tablet': '640px',
// => @media (min-width: 640px) { ... }
'laptop': '1024px',
// => @media (min-width: 1024px) { ... }
'desktop': '1280px',
// => @media (min-width: 1280px) { ... }
},
}
}
Your responsive modifiers will reflect these custom screen names, so using them in your HTML would now look like this:
<div class="grid grid-cols-1 tablet:grid-cols-2 laptop:grid-cols-3 desktop:grid-cols-4">
<!-- ... -->
</div>